Elixir
Anonymous Functions & Lambdas: Elixir = Ruby
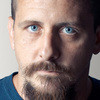
This week in the Elixir Cauldron we are going to compare Elixir's anonymous functions to Ruby's lambda expressions. Although we don't think of Ruby as a functional language, there are features of Ruby that resemble its functional cousins, including its use of lambda expressions.
Let's look at examples in both languages. First, define an anonymous function in Elixir and then look at the Ruby equivalent.
iex(1)> say = fn() -> IO.puts "Hello" end
iex(2)> say.()
Hello
:ok
Here is the same functionality replicated in Ruby:
irb(main):001:0> say = -> { puts "Hello" }
irb(main):002:0> say.()
Hello
=> nil
Those were oversimplified examples, but they do illustrate the syntactical similarities in the two languages. The similarities don't stop there.
Like other functional languages, Elixir functions are first class citizens. What does that mean? It means that functions not only yield values but they can also be assigned to variables, passed as arguments, or returned from other functions. We've seen functions assigned to variables, so how does passing as an argument or returning a function compare?
To see this in action, we will define a function that accepts a function as an argument and executes it:
defmodule Simple do
def call(func) do
func.()
end
end
Now in iex
call the function, passing an anonymous function as an argument:
iex(1)> Simple.call fn() -> IO.puts "Hello" end
Hello
:ok
How would that be implemented in Ruby and irb
?
class Simple
def self.call(func)
func.()
end
end
irb(main):001:0> Simple.call -> { puts "Hello" }
Hello
=> nil
What about returning a function from another function?
defmodule Simple do
def return do
fn() -> IO.puts "Hello" end
end
end
Now call the function from iex
:
iex(1)> say = Simple.return
iex(2)> say.()
Hello
:ok
And again in Ruby and irb
:
class Simple
def self.return
-> { puts "Hello" }
end
end
irb(main):001:0> say = Simple.return
irb(main):002:0> say.()
Hello
=> nil
These are simple examples but their purpose is to illustrate the similarities between the two languages. Uncovering syntactical similarities can ease a transition between languages.
Next week, we will expand our view of Elixir functions by addressing a feature Ruby doesn't include, pattern matching.