React Javascript
Writing Prettier JavaScript in Vim
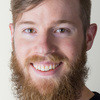
JavaScript is an incredibly powerful and fickle language. In the face of quickly evolving frameworks and tooling, it can be cognitively challenging to grapple even with a familiar codebase. Even just having one less thing to think about goes a long way in helping us deal with the rest. Figuring out how to format each line of code is accidental complexity and one less thing we ought to think about.
As Brian Dunn put it, "Once you stop formatting your code, life becomes beautiful." And so does your code, but without any of the effort.
This is where Prettier comes in.
At Hashrocket, Vim is an essential part of our development tooling.
neoformat
makes it easy to
incorporate prettier
into our existing workflow. As you can see in the
above gif, Prettier formats the file on save.
Here is how we set it up.
Step 1
Install prettier
if you haven't already.
$ yarn global add prettier
(or npm install -g prettier
if you prefer)
Step 2
Install the neoformat
vim plugin.
" ~/.vimrc
Plug 'sbdchd/neoformat'
This plugin is a general-purpose formatter that can be configured to work across many filetypes. You can check out the repository README for the full list of supported filetypes.
Step 3
Next we need to configure Neoformat to use Vim's formatprg
as its
formatter.
let g:neoformat_try_formatprg = 1
This way in the following step we can specify some options for prettier
.
Step 4
We want neoformat
to be configured specifically to format our JavaScript
files with prettier
using a few configuration flags of our choosing. We
can set up an autogroup in our ~/.vimrc
file for that.
" ~/.vimrc
augroup NeoformatAutoFormat
autocmd!
autocmd FileType javascript setlocal formatprg=prettier\
\--stdin\
\--print-width\ 80\
\--single-quote\
\--trailing-comma\ es5
autocmd BufWritePre *.js Neoformat
augroup END
The FileType
line sets prettier
as the format program whenever a
javascript
file is encountered. formatprg
will provide the file contents
to prettier
via stdin
, so the --stdin
flag tells prettier
to expect
as much. The --print-width
flag tells prettier
to keep lines under 80
characters. And so on. The rest of the details are in the
Options section of the
Prettier README.
Step 5
We work on a lot of React.js projects, so having support for JSX is
essential. The time it takes to reformat long lines of JSX means that we can
feel the time savings quite acutely when prettier
does the formatting for
us.
To accommodate React, we can update our existing autogroup by adding support
for the jsx
filetype. This requires that you already have the
vim-jsx
plugin installed.
" ~/.vimrc
augroup NeoformatAutoFormat
autocmd!
autocmd FileType javascript,javascript.jsx setlocal formatprg=prettier\
\--stdin\
\--print-width\ 80\
\--single-quote\
\--trailing-comma\ es5
autocmd BufWritePre *.js,*.jsx Neoformat
augroup END
All Set
Give this setup a try and enjoy some consistent, well-formatted code. Forget about the formatting and focus on the function.
Shoutout to Dorian Karter for introducing me to Prettier in Vim.
Cover photo by JOHN TOWNER on Unsplash