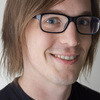
Here's the thing: I've settled on a group of simple, practical helper methods that I've ended up using time and time again to reliably generate useful dummy data. It's made my life easier, and I bet it could do the same for you. My use of Faker alongside our UI Controller process (detailed here: part 1, part 2) has developed to the point that I have deemed it worthy of one more post on the topic.
So, here's a recent use case. I needed to output a category management interface. This would basically be a list of category checkboxes with subcategory checkboxes, e.g.
%ul
%li
%label
%input(type="checkbox")
Category Label
%ul
%li
%label
%input(type="checkbox")
Subcategory Label
Pretty straightforward, right? Well, the issue we were having is that we were unsure how the interface we were designing would handle long category names or large numbers of subcategories (there's a design post in there somewhere about designing interfaces that elegantly handle odd data, but this post is about the coding side of the process).
So here's where Faker helps a ton. I have a couple of cute methods that do the randomization work for us. Here's one that gives us any number in a range (e.g. around(1..5)
):
def around(range)
range.to_a.sample
end
And here's one that gives us either a number or range of filler words:
def lorem_words(num)
num = around(num) if num.is_a?(Range)
Faker::Lorem.words(num).join(' ').html_safe
end
Lastly, this particular interface would hide & show category sections based on whether the checkbox was checked, so I needed to output either true or false. I could just say around([true, false])
but that's a common enough need and these helpers are for readability & ease of typing, so I'll just have a method for it.
def coinflip
around([true, false])
end
So now, with very little extra effort, we can test our UI against a wide range of possible values:
%ul
- around(4..20).times do
%li
%label
%input(type="checkbox" checked=coinflip)
= lorem_words(1..3)
%ul
- around(3..8).times do
%li
%label
%input(type="checkbox" checked=coinflip)
= lorem_words(1..3)
Hooray! This is some entry-level Ruby, I know, but it's infinitely more valuable than static dummy content, and using techniques like this has allowed me to catch potential layout issues long before they're uncovered by real data after implementation.
Here's a gist of the UI Helper as it currently stands – and many thanks to a number of fellow Rocketeers who know far more about Ruby than I do and helped make the whole thing much more elegant. I hope it's as useful for you as it has been for me.