Ruby
Test with a Sign In Backdoor
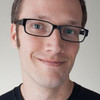
Did you know that almost every scenario in your acceptance test suite has to sign the user in? Did you know that you are crazily overtesting your sign in page as a result? It doesn't have to be this way.
Typically a login step looks something like this:
Given /^I am signed in$/ do
visit '/sign_in'
fill_in 'Email', with: user.email
fill_in 'Password', with: 'password'
click_button 'Sign In'
end
It looks reasonable but every single scenario is requesting and rendering the same page, filling out the same fields, and submitting them. Signing in through the browser is necessary to establish the session, but it doesn't need to be this painful.
You can implement a backdoor to take this down to a single page request with no rendering. Even better, you can include it in features/support
so you don't open yourself up to any security vulnerabilities.
features/support/sign_in_backdoor.rb
class UserSessionsController
def backdoor
sign_in(User.find_by_email(params[:email]))
redirect_to :root
end
end
MyRailsApp::Application.routes.tap do |routes|
routes.disable_clear_and_finalize = true
routes.draw do
match 'backdoor', to: 'user_sessions#backdoor'
end
end
Then you can update your step to look like this:
module SessionStepMethods
def sign_in(user)
visit "/backdoor?email=#{user.email}"
end
end
World(SessionStepMethods)
Given /^I am signed in$/ do
sign_in(@user)
end
If you have 500 scenarios in your suite, you just saved 500 full page requests. Great work!