Elixir
Silence Logger Messages in ExUnit
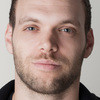
Logger messages can be a distracting noise when running multiple tests. Fortunately ExUnit provides some great tooling to prevent unwanted logger messages when running your tests.
Take this plug route for example:
defmodule MyApp.Router do
require Logger
use Plug.Router
use Plug.Debugger, otp_app: :my_app
alias MyApp.SlackResponder
plug(Plug.Logger)
plug(Plug.Parsers,
parsers: [:json, :urlencoded],
json_decoder: {Jason, :decode!, [[keys: :atoms]]}
)
plug(:match)
plug(:dispatch)
post "/" do
response = SlackResponder.respond(conn.params)
send_resp(conn, 200, response)
end
match _ do
Logger.debug("Got a request I don't understand #{inspect(conn)}")
send_resp(conn, 404, "not_found")
end
end
And the test:
test "returns 404 when url doesn't match" do
conn =
conn(:post, "/bogus_url", %{"text" => "hi"})
|> Router.call(Router.init([]))
assert conn.state == :sent
assert conn.status == 404
end
Running this test will produce:
To mute the logger send the capture_log
options to ExUnit.start
in test/test_helper.exs
:
ExUnit.start(capture_log: true)
Now upon re-running the test the logs will not show up, unless the test failed! What a great feature!!!
Passing example:
Failure example:
You can also control this feature more granularly by tagging individual tests:
@tag capture_log: true # Default is `false`
test "returns 404 when url doesn't match" do
# ...
end
To learn more: https://hexdocs.pm/ex_unit/ExUnit.html#configure/1
Or if you are inside of iex
:
iex> require ExUnit
iex> h ExUnit.configure
Photo by Kristina Flour on Unsplash