Javascript
5 JavaScript Object Destructuring Tricks
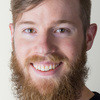
The ES6 destructuring syntax is quite flexible. It allows us to do a lot of nice things with objects and arrays that in the past would have taken many distracting lines of code.
Let's look at five handy destructuring tricks for objects.
Trick #1
Remove an item from an object
// const data = { a: 1, b: 2, thingToRemove: "bye" };
const { thingToRemove, ...rest } = data;
console.log(rest); // { a: 1, b: 2 }
This is a concise way of getting a new copy of an object with a specific
key/value pair removed. Note that it is immutable and you are free to
specify as many keys as you'd like separated from the ...rest
object.
Trick #2
Rename things in a destructuring
// const data = { coordinates: { x: 5, y: 6 }};
const { x: xCoord, y: yCoord } = data.coordinates;
console.log(xCoord, yCoord); // 5, 6
It is not always desirable or possible to adjust naming within an object upstream. If we need different naming locally, we can do this inline with our destructuring. The colon syntax allows us to do this with specific keys.
Trick #3
Nested Destructuring
// const data = { coordinates: { x: 5, y: 6 }};
const { coordinates: { x, y }} = data;
console.log(x, y); // 5, 6
We can get at key/value pairs in a single destructuring regardless of how they are nested. This again allows us to concisely access the data we want without having to make any upstream adjustments.
This can of course be combined with Trick #2:
// const data = { coordinates: { x: 5, y: 6 } };
const { coordinates: { x: xCoord, y: yCoord } } = data;
console.log(xCoord, yCoord); // 5, 6
Fancy!
Trick #4
Destructuring In Function Arguments
All the things we did above -- we can do those in the argument part of a function declaration.
const myFunction = ({ coordinates: { x: xCoord, y: yCoord } }) => {
console.log('Coords:', xCoord, yCoord);
};
We've accessed and renamed nested values all in the function declaration. The body of the function can be focused strictly on the logic.
Trick #5
Non-default Imports As Named Object
Consider a growing list of imports that starts to look like this:
import {
rootPath,
blogPath,
aboutUsPath,
teamPath,
pricingPath,
contactPath,
signInPath,
signOutPath,
} from '../routes';
It's already unwieldy and its bound to get worse. This import destructuring syntax allows us to tame those imports.
import { * as routes } from '../routes';
console.log(routes.rootPath); // '/'
console.log(routes.blogPath); // '/blog'
This can be a nice way to not have to explicitly import dozens of things The tradeoff is that your compiler will no longer be able inform you if you are referencing an undefined import.
Conclusion
These five object destructuring tricks allow us to write more concise, direct code. I can attest to the usefulness of these tricks -- quickly browsing through a few files of a React app I've been maintaining for over a year reveals each of these employed in a variety of contexts. Give them a try, I think you'll find that it makes JavaScript even more fun to write.
Cover Photo Credit: Franck V.