Vim
Validating JSON File Contents With Your Text Editor
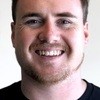
I recently ran into some problems with my editor config around vim-projectionist
. Of course the fix was super easy - there was invalid JSON in my .projections.json
file. This vim plugin is a core part of our workflow at Hashrocket, so I wanted to figure out a way to prevent this from happening again. So today, I want to propose 2 solutions that will help you easily catch those pesky JSON errors in your editor.
1. Install a JSON language server
This may seem obvious, but I'm sure it can be overlooked during initial setup. Catching the errant trailing comma or other issues can be surfaced very quickly, while you're editing the file.
If you're using VSCode, there's vscode-json-languageserver. Just add it to your plugins and you're good to go.
If you're using Neovim and nvim-lsp, there's jsonls which you can configure in your init.lua
.
2. Payload Validator Script
The alternative to either of these is to write a small script to validate your .projections.json
payload when present and give feedback within your editor.
Let's assume you are using nvim
without LSP or other validation plugins. Here's something I whipped up rather quickly -
First, I made a ruby script to load and parse the projections file when present. If there's a projections file with JSON errors, we exit with code 1, otherwise 0.
require "json"
class BasicObject
def blank?
self.nil? || self.to_s.strip == ""
end
end
project_dir = ARGV[0]
custom_projections = File.join(project_dir, ".projections.json")
if project_dir.blank? || !File.exist?(custom_projections)
exit 0
end
begin
JSON.parse(File.read(custom_projections))
exit 0
rescue JSON::ParserError
puts "Invalid JSON detected in .projections.json"
exit 1
end
You can manually run the script from the command line to test things out.
ruby projections_check.rb ~/Hashrocket/hashrocket.com
Next, we setup a lua function to run our script and check the exit code. If it's non-zero, then we notify the user with the error message.
local function run_script_with_cwd(command, cwd, error_message)
-- Run the command and capture the exit code
local exit_code = os.execute(command)
-- Check the exit status
if exit_code ~= 0 then
vim.schedule(function()
vim.notify(
error_message .. " " .. exit_code,
vim.log.levels.ERROR
)
end)
end
end
-- Check if a projections file is defined and valid JSON
local config_dir = vim.fn.stdpath("config")
local projections_check_script = config_dir .. "/projections_check.rb"
run_script_with_cwd(
string.format("ruby %s %s", projections_check_script, vim.fn.getcwd()),
vim.fn.getcwd,
"Custom .projections file is invalid"
)
And that's it! To test out the whole thing, I setup some invalid json in my projections file. The next time we open nvim with invalid projections, we are notified that the projections file is invalid.
Happy Hacking!