Ruby
How to Make a Rails 5 App API-Only
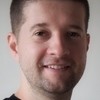
This blog post lists the steps to follow when converting your regular Rails 5 app to api only mode. Rails 5 has released this feature and the main purpose is to be a lighter version of Rails. This means less middleware on the stack and less modules included in controllers. Api only mode is optimized to serve json content. This way your app will fit better within frontend/backend architectures.
If your app is still on Rails 4 read this blog post.
Some historical context
So this feature was already part of Rails code very briefly on March 2012 (added and removed). By that time the feature was suppose to be activated with --http
, like: rails new my-backend --http
.
Afterwards it was transformed into a gem rails-api and heavily used by a lot of applications.
Finally, after more than 3 years, this code was merged into Rails code. In order to setup a new project with api mode:
rails new my-rails-api --api
Migrating to Rails 5 API app from a traditional app
If your Rails app is upgraded already to Rails 5.0 and it is an api only, with no HTML rendering or browser stuff, follow these steps:
1 - Define api only mode
Add config.api_only = true
to config/application.rb
file:
# config/application.rb
module MyRails5Api
class Application < Rails::Application
config.api_only = true
end
end
With this configuration your Rack middleware will be shortened. On APIs you don't need to have Cookies or Flash middlewares.
2 - Remove unused web gems
Some gems that comes with Rails for web mode are not needed when api_only
mode is on. Here some examples of gems you may want to remove:
coffee-rails
jquery-rails
sass-rails
uglifier
turbolinks
web-console
3 - Files/Folders can be remove as well:
You won't need these files and folders anymore:
app/assets/
lib/assets/
vendor/assets/
app/helpers/
app/views/layouts/application.hmtl.erb
You should have just Mailer views on your app/views
folder.
4 - ApplicationController Inheritance
Change your ApplicationController
to inherit from ActionController::API
:
# app/controller/application_controller.rb
class ApplicationController < ActionController::API
end
This will not include some browser related modules for all of your controllers.
You'll also need to remove: protect_from_forgery with: :exception
for the same file.
5 - Generators
From now on your generators will identify that you have set your api_only
mode and then will generate API like controllers. Also there will be no more views being generated.
6 - JSON serializer
You can choose your preferred json serializer solution for that. Rails has an agnostic solution for that and relies on the implementation of to_json
method. The top 2 solutions are: jbuilder and active_model_serializers.
7 - CORS
If your backend and frontend has different domains you'll need to enable CORS (cross-origin HTTP request). At this point you probably know that. Rails 5 now is "suggesting" to use rack-cors
gem by letting commented that on new generated projects. Here it is a simple example:
# config/initializers/cors.rb
Rails.application.config.middleware.insert_before 0, "Rack::Cors" do
allow do
origins 'localhost:4200'
resource '*',
headers: :any,
methods: %i(get post put patch delete options head)
end
end
Conclusion
Rails is well known for being a great tool for building web applications, and also for APIs. It took a long time, but now Rails 5 has brought the same Rails way to develop in the API world and this is amazing.