Javascript
The Impact of the Exclamation Point in TypeScript
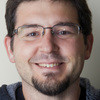
In TypeScript, a language built on top of JavaScript to add static typing, the exclamation point !
is not just a symbol of excitement or emphasis as it is in English; it plays a critical role in the type system. This article delves into the significance of the exclamation point and how it influences TypeScript's static analysis of code.
Non-Null Assertion Operator
The primary use of the exclamation point in TypeScript is as the "non-null assertion operator." In a language that embraces types to reduce runtime errors, TypeScript introduces a way to tell the compiler, "trust me, I know what I'm doing." The !
operator, when appended to the end of an expression, tells TypeScript that the value is not null or undefined.
Here’s a quick example:
let maybeString: string | null = document.querySelector('.myInput').value;
console.log(maybeString.length); // Error: Object is possibly 'null'.
console.log(maybeString!.length); // No error
The first console log will flag a potential error in this code snippet because maybeString
could be null
. However, when we append !
, we assert that we know maybeString
will have a value, thus negating the null check and preventing the TypeScript error.
Why Use It?
The question arises: why would you need to bypass TypeScript's safety net? In specific scenarios, a developer is more aware of the context than TypeScript can be. For instance, you might know that a DOM element exists because it's statically in the HTML, but TypeScript can't assume that as it checks the code without DOM context. In such cases, !
allows for more flexible and assertive coding.
The Risks
The non-null assertion operator is powerful but should be used judiciously. Overuse or misuse can undermine the very purpose of TypeScript, which is to catch errors at compile time rather than at runtime. If you're wrong about your assertion, you will have silenced TypeScript's warning only to encounter a potential runtime error.
Best Practices
- Safety First: Use the non-null assertion operator only when you have absolute certainty that a value will not be null or undefined.
- Runtime Guarantees: Whenever possible, ensure runtime guarantees for the presence of values rather than relying on static assertions.
- Code Smells: Frequent use of
!
might indicate a code smell. Consider if your code structure can be refactored to better utilize TypeScript's type system.
Conclusion
The exclamation point in TypeScript is a subtle but powerful tool. It exemplifies TypeScript's flexible approach to typing, allowing developers to override the compiler's strictness when they have specific knowledge about the values in their code. However, with great power comes great responsibility. It should be used sparingly and wisely, as an incorrect assertion can lead to the very bugs TypeScript aims to prevent. When used correctly, the !
operator can be a declaration of confidence in the face of potential nullability, allowing seamless integration of static typing into the dynamic world of JavaScript.